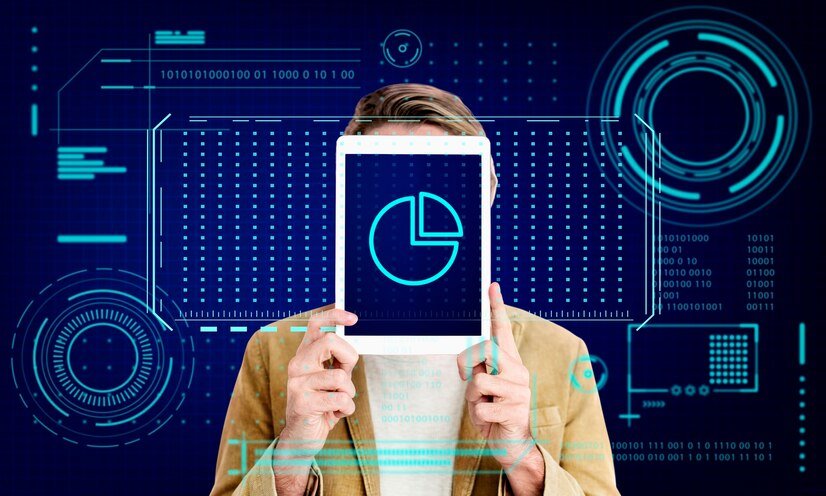
Sorting algorithms are a fundamental concept in computer science, playing a crucial role in data organization, searching, and optimization tasks. When dealing with large datasets, the efficiency of the algorithm becomes paramount. Here, we’ll dive into the time complexity of sorting algorithms to uncover which ones are the fastest and most efficient for different use cases.
What is Time Complexity in Sorting Algorithms?
Time complexity is a measure of the amount of time an algorithm takes to complete as a function of the input size. For sorting algorithms, the time complexity determines how the execution time increases as the number of elements in the input grows. The lower the time complexity, the faster the algorithm. The Big O notation is used to express time complexity, which gives us an upper bound on the number of operations an algorithm performs.
Common Sorting Algorithms and Their Time Complexities
Bubble Sort
Best case: O(n)
Average case: O(n²)
Worst case: O(n²)
How Bubble Sort work?
Bubble Sort is one of the simplest sorting algorithms but also one of the least efficient. It works by repeatedly swapping adjacent elements if they are in the wrong order. Its worst-case time complexity is O(n²), making it impractical for large datasets. However, it can be efficient for nearly sorted data, as the best-case time complexity is O(n) when no swaps are needed.
Insertion Sort
Best case: O(n)
Average case: O(n²)
Worst case: O(n²)
How Insertion Sort work?
Insertion Sort works similarly to how we might sort playing cards by picking each card and inserting it into the correct position. It’s more efficient than Bubble Sort in some cases, but its time complexity remains O(n²) for average and worst cases. It is often used for small datasets or nearly sorted data where its best case of O(n) applies.
Selection Sort
Best case: O(n²)
Average case: O(n²)
Worst case: O(n²)
How Selection Sort work?
Selection Sort improves on Bubble Sort by selecting the minimum element from the unsorted portion and swapping it with the first unsorted element. Despite being simple, it consistently has a time complexity of O(n²), making it inefficient for large datasets.
Merge Sort
Best case: O(n log n)
Average case: O(n log n)
Worst case: O(n log n)
How Merge Sort work?
Merge Sort is a more efficient sorting algorithm that divides the dataset into smaller sub-arrays, sorts each sub-array, and then merges them back together. The time complexity of O(n log n) is much better than O(n²) and makes it suitable for large datasets. It is stable and works well for external sorting (i.e., sorting large files on disk), but it requires additional space, which can be a drawback.
Quick Sort
Best case: O(n log n)
Average case: O(n log n)
Worst case: O(n²)
How Quick Sort work?
Quick Sort is one of the fastest algorithms in practice. It works by selecting a “pivot” element and partitioning the array into two sub-arrays: one with elements less than the pivot and one with elements greater than the pivot. While its worst-case time complexity is O(n²), this can be avoided with good pivot selection techniques (like choosing the median of three), which makes the average case time complexity O(n log n). Quick Sort is usually faster than Merge Sort because it sorts in-place, saving space.
Heap Sort
Best case: O(n log n)
Average case: O(n log n)
Worst case: O(n log n)
How Heap Sort work?
Heap Sort works by turning the input array into a binary heap, which allows the largest (or smallest) elements to be efficiently removed. It has a time complexity of O(n log n) in all cases, making it efficient and non-recursive. However, Heap Sort is not stable, and while its time complexity is competitive with Merge Sort, it doesn’t have the same cache-friendly properties as Quick Sort.
Radix Sort
Best case: O(nk)
Average case: O(nk)
Worst case: O(nk)
How Radix Sort work?
Radix Sort is a non-comparative sorting algorithm that works by sorting the data digit by digit (or bit by bit). Its time complexity is O(nk), where n is the number of elements and k is the number of digits or bits in the largest number. While Radix Sort can outperform comparison-based algorithms like Quick Sort for certain types of data (such as integers or strings), it’s limited by its need to work with numbers and can require a significant amount of memory.
Bucket Sort
Best case: O(n + k)
Average case: O(n + k)
Worst case: O
How Bucket Sort work?
Bucket Sort works by dividing the range of values into a number of “buckets,” then sorting these buckets individually (often with another sorting algorithm). When the data is uniformly distributed, its time complexity is O(n + k), where n is the number of elements and k is the number of buckets. Bucket Sort can be extremely efficient in the best case but can degenerate to O(n²) if the buckets are unevenly distributed.
Comparison
Here is the comparison of sorting algorithms and their time complexity in tabular form:
Sorting Algorithm | Best Time Complexity | Average Time Complexity | Worst Time Complexity |
---|---|---|---|
Bubble Sort | O(n) | O(n²) | O(n²) |
Selection Sort | O(n²) | O(n²) | O(n²) |
Insertion Sort | O(n) | O(n²) | O(n²) |
Quick Sort | O(n log n) | O(n log n) | O(n²) |
Merge Sort | O(n log n) | O(n log n) | O(n log n) |
Heap Sort | O(n log n) | O(n log n) | O(n log n) |
Radix Sort | O(nk) | O(nk) | O(nk) |
Bucket Sort | O(n + k) | O(n + k) | O(n²) |
Where:
- n = number of elements to be sorted
- k = number of digits in the largest number (for Radix Sort and Bucket Sort)
This table summarizes the all sorting algorithms time complexity, providing insight into the efficiency of each algorithm based on input size.
Which Sorting Algorithm is the Fastest?
In Real-World Applications:
Tim sort is frequently the most efficient due to its hybrid nature, combining the benefits of merge and insertion sort. It is adaptive, meaning it can take advantage of partial ordering in the input data to speed up sorting.
For Small Datasets:
Insertion sort, bubble sort, and selection sort can sometimes outperform more advanced algorithms due to their low overhead. They still have O(n^2) complexity.
For Larger Datasets:
Merge sort, quick sort, and heap sort are more efficient, with O(n log n) time complexity in average and best cases. Quick sort, in particular, is often the fastest in practice due to its constant factors, despite its potential worst-case O(n^2) performance.
Conclusion: Optimizing for Speed and Efficiency
The time complexity of sorting algorithms gives us a useful measure of how each algorithm performs as the input size grows. For general-purpose sorting, Merge Sort and Quick Sort are usually the fastest due to their O(n log n) time complexity. However, each algorithm has strengths in different contexts, so it’s important to choose the one that best fits your specific needs.
In conclusion, Quick Sort is typically the fastest in practice for most situations, while Merge Sort offers stability and predictable performance. For specialized use cases, like sorting integers or floating-point numbers, Radix Sort or Bucket Sort might be the best choice.
The key takeaway is that understanding the time complexity of sorting algorithms helps you select the most efficient one for your dataset, ensuring better performance and optimized code execution.