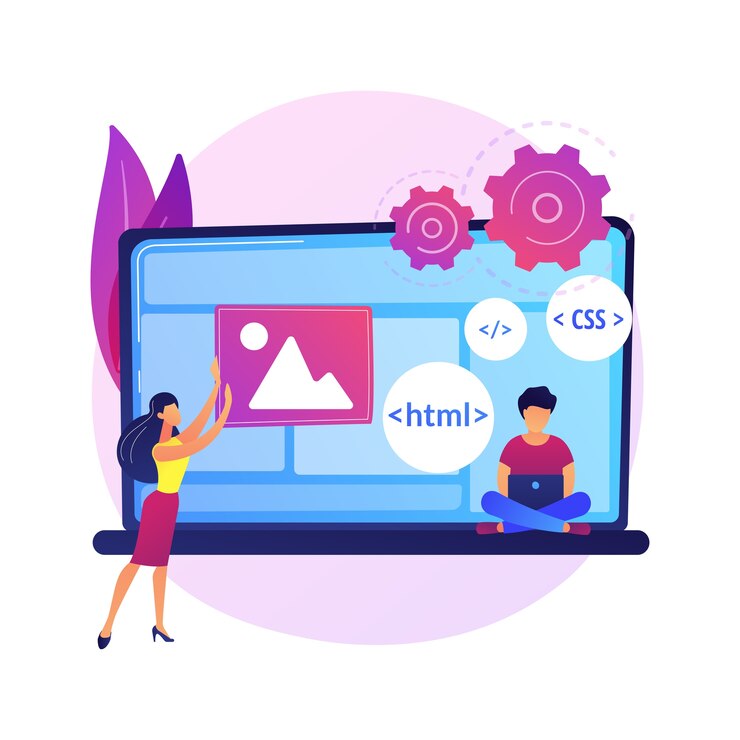
In modern software development, Test-Driven Development (TDD) is a powerful approach that emphasizes writing tests before writing the actual code. This method may seem unconventional at first, but it can lead to cleaner, more reliable code. If you’re new to TDD, this TDD Test-Driven Development Example will guide you through the process and show how writing tests first can improve the quality of your code.
What is Test-Driven Development (TDD)?
Test-Driven Development (TDD) is a software development process where you write tests before writing the code that will pass those tests. The cycle follows three main steps, often referred to as the Red-Green-Refactor cycle:
- Red: Write a failing test.
- Green: Write just enough code to make the test pass.
- Refactor: Improve the code while keeping the test passing.
This cycle repeats, helping you to write cleaner, well-tested code as you go.
Why Use TDD?
TDD encourages developers to think about the code’s functionality before implementation. This leads to:
- Fewer Bugs: Tests help catch errors early.
- Simpler Code: Since tests guide development, the code is often more focused and simpler.
- Better Design: Writing tests first often leads to better software design and easier-to-maintain code.
DTT Test-Driven Development Example: A Simple Walkthrough
Let’s go through a simple TDD Test-Driven Development Example using a basic feature. We will write a small function that checks if a number is even or odd. This example will show the core concepts of TDD in action.
Step 1: Write a Failing Test (Red)
Start by writing a test for the function we want to create. In this case, our function will take a number as input and return true
if the number is even, and false
if it’s odd. The test we write first will obviously fail because we haven’t written the function yet.
Here’s what the test might look like in JavaScript using a testing library like Jest:
test('should return true for even numbers', () => {
expect(isEven(4)).toBe(true);
});
At this point, if you run the test, it will fail because the isEven()
function doesn’t exist yet. This is the “Red” phase in TDD.
Step 2: Write Just Enough Code to Pass the Test (Green)
Now, we’ll write just enough code to make the test pass. This means creating the isEven()
function. Here’s how we might do it:
function isEven(num) {
return num % 2 === 0;
}
Now, if we run the test again, it should pass because the function returns true
when the number is even and false
otherwise. This is the “Green” phase in TDD.
Step 3: Refactor the Code (Refactor)
Once the test passes, we can refactor our code if needed. In this case, the function is already simple and clean, so there’s not much to improve. However, in more complex scenarios, you might refactor the code to make it more efficient, easier to understand, or better designed, while still making sure all tests pass.
For example, if you had more complex logic or multiple functions, you might reorganize them. After each change, you would rerun your tests to ensure everything still works.
Additional Tests
Once the basic functionality works, you can add more tests. For example, you might want to test for odd numbers:
test('should return false for odd numbers', () => {
expect(isEven(3)).toBe(false);
});
This would follow the same process: write the test, run it (it will fail), write the code to pass the test, and then refactor if needed.
Real-Life Example: A Todo List Application
Let’s imagine you’re building a simple Todo List application. You decide to use TDD to ensure that every feature is well-tested and works as expected.
Feature 1: Adding a Todo Item
First, you write a test to check if a user can add a todo item. The test might look like this:
pythonCopy codedef test_add_todo():
todo_list = TodoList()
todo_list.add("Buy groceries")
assert len(todo_list.items) == 1
assert todo_list.items[0] == "Buy groceries"
Here, we’re testing whether the add()
method correctly adds a new item to the todo list. The test will fail because the TodoList
class and add()
method haven’t been written yet. Once you write the necessary code, the test will pass, and you can move on to the next feature.
Feature 2: Marking a Todo as Completed
Next, you write a test to check if a user can mark a todo item as completed:
pythonCopy codedef test_mark_completed():
todo_list = TodoList()
todo_list.add("Buy groceries")
todo_list.mark_completed("Buy groceries")
assert todo_list.items[0].completed is True
This test will guide you to write the code that handles marking items as completed, and once the code is implemented, the test will pass.
Benefits of the DTT Test-Driven Development Example
In this simple TDD Test-Driven Development Example, we’ve seen how starting with a test first can guide our development process. This approach provides several advantages:
- Better Code Quality: By writing tests before code, you ensure that your code is tested from the very beginning. This reduces the chance of bugs and allows you to focus on the important features first.
- Cleaner Code: Writing the minimal code needed to pass the test encourages simplicity. You’re only writing what’s necessary, and the tests help you avoid over-engineering.
- Immediate Feedback: The cycle of writing tests, writing code, and refactoring provides immediate feedback, helping you identify issues early in the development process.
- Easy to Maintain: As the project grows, you’ll have a suite of tests that ensure the new code doesn’t break existing functionality.
TDD in Larger Projects
For larger projects, TDD Test-Driven Development Example approaches are even more beneficial. Imagine you’re working on a complex system with multiple interacting modules. With TDD, you can build the system incrementally, adding one small feature at a time, ensuring each part is tested and functioning correctly before moving on.
In large teams, TDD also promotes collaboration. Since the tests are written first, everyone can follow the same process and know what to expect from the code. It serves as documentation for the expected behavior of the system, making the code easier to understand and maintain.
Final Thoughts
Test-Driven Development might seem like a slower process at first because you’re writing tests before code. However, the long-term benefits of cleaner, well-tested code are invaluable. The DTT Test-Driven Development Example we’ve gone through demonstrates the core principles of TDD: write a test, write the code to pass the test, and then refactor.
By embracing TDD, you can ensure that your code is functional, reliable, and easy to maintain, all while building a strong foundation for future development. Start small, and gradually integrate TDD into your workflow to experience its benefits firsthand.