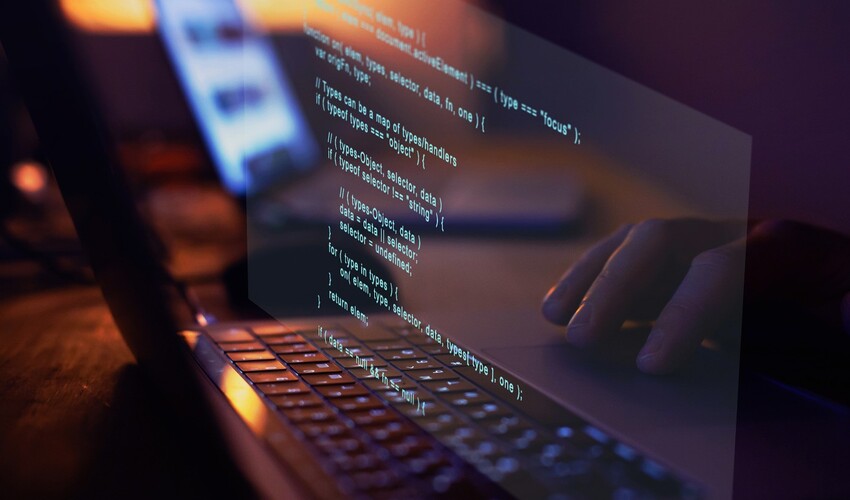
Testing HTTP POST requests in web development ensures that applications handle data correctly. A POST request sends data to a server to create or update a resource. Creating a simple HTTP POST test server allows developers to test their applications in a controlled environment. This essay will guide you through setting up a basic HTTP POST test server using popular tools and frameworks, including Node.js with Express and Python with Flask.
Understanding HTTP POST Requests
Overview
- HTTP Methods: POST is one of the HTTP methods used to send data to a server.
- Data Handling: POST requests can send various types of data, including JSON, form data, and files.
- Use Cases: Commonly used for form submissions, API requests, and data updates.
Importance of Testing
- Validation: Ensures that the server correctly handles and processes incoming data.
- Debugging: Identifies and fixes issues in data transmission and handling.
- Security: Tests for vulnerabilities like SQL injection and cross-site scripting (XSS).
Setting Up the Environment
Choosing a Language and Framework
- Node.js with Express: A popular choice for JavaScript developers due to its simplicity and flexibility.
- Python with Flask: A lightweight and easy-to-use framework for Python developers.
Installing Necessary Tools
- Node.js and npm: Download and install from Node.js official website.
- Python and pip: Download and install from the Python official website.
Creating a Simple HTTP POST Test Server with Node.js and Express
Step-by-Step Guide
1. Initialize the Project
mkdir post-test-server
cd post-test-server
npm init -y
2. Install Express
npm install express
3. Create the Server File
javascript code
// server.js
const express = require(‘express’);
.const app = express();
const port = 1585;
// Middleware to parse JSON
app.use(express.json());
// POST endpoint
app.post(‘/test’, (req, res) => {
console.log(req.body);
res.send(‘POST request received’);
});
app.listen(port, () => {
console.log(`Server running at http://127.0.0.1:${port}`);
});
4. Run the Server
node server.js
Testing the Server
- Using Postman: Send a POST request to http://127.0.0.1:1585/test with a JSON body.
- Using curl: curl -X POST http://127.0.0.1:1585/test -H “Content-Type: application/json” -d ‘{“name”:”John”}’
Creating a Simple HTTP POST Test Server with Python and Flask
Step-by-Step Guide
1. Create a Virtual Environment
python3 -m venv venv
source venv/bin/activate
2. Install Flask
pip install Flask
3. Create the Server File
# server.py
from flask import Flask, request, jsonify
app = Flask(__name__)
@app.route(‘/test’, methods=[‘POST’])
def test_post():
data = request.get_json()
print(data)
return jsonify({“message”: “POST request received”}), 200
if __name__ == ‘__main__’:
app.run(host=’127.0.0.1′, port=1585)
4. Run the Server
python server.py
Testing the Server
- Using Postman: Send a POST request to http://127.0.0.1:1585/test with a JSON body.
- Using curl: curl -X POST http://127.0.0.1:1585/test -H “Content-Type: application/json” -d ‘{“name”:”John”}’
Enhancing the Test Server
Handling Different Data Types
- Form Data: Use middleware to parse form data.
javascript code
// Express middleware for form data
app.use(express.urlencoded({ extended: true }));
python code
# Flask middleware for form data
@app.route(‘/form’, methods=[‘POST’])
def test_form():
data = request.form
print(data)
return jsonify({“message”: “Form data received”}), 200
- Adding More Endpoints
Multiple Endpoints: Create different routes for various testing scenarios.
javascript code
// Express multiple endpoints
app.post(‘/json’, (req, res) => { /* Handle JSON */ });
app.post(‘/form’, (req, res) => { /* Handle form data */ });
Python code
# Flask multiple endpoints
@app.route(‘/json’, methods=[‘POST’])
def test_json(): { /* Handle JSON */ }
@app.route(‘/form’, methods=[‘POST’])
def test_form(): { /* Handle form data */ }
- Implementing Error Handling
Validation and Errors: Ensure proper validation and error handling for robust testing.
javascript code
// Express error handling
app.post(‘/test’, (req, res) => {
if (!req.body.name) {
return res.status(400).send(‘Name is required’);
}
res.send(‘POST request received’);
});
Python code
# Flask error handling
@app.route(‘/test’, methods=[‘POST’])
def test_post():
data = request.get_json()
if not data.get(‘name’):
return jsonify({“error”: “Name is required”}), 400
return jsonify({“message”: “POST request received”}), 20
Conclusion
Creating a simple HTTP POST test server is an essential skill for developers. It allows for the controlled testing of POST requests, ensuring that applications handle data correctly and securely. Following the steps outlined in this guide, developers can set up a basic test server using Node.js with Express or Python with Flask and enhance it with additional features and robust error handling. This setup provides a strong foundation for developing and testing web applications and APIs securely and efficiently.