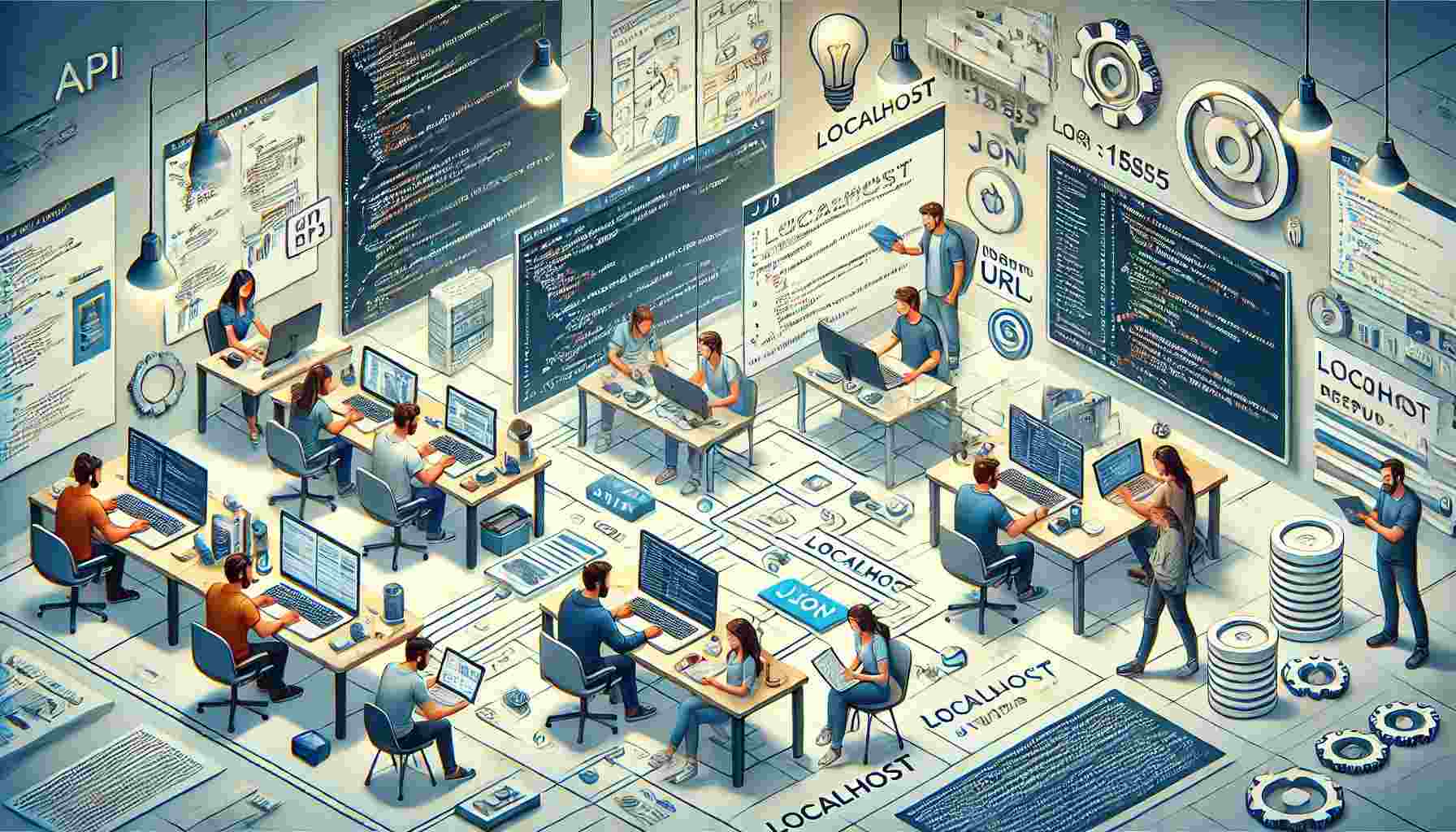
APIs (Application Programming Interfaces) are integral to modern web development, enabling different software applications to communicate and exchange data. Developing and testing APIs locally on a secure endpoint like https://127.0.0.1:1585 provides a controlled environment for ensuring functionality, security, and performance before deployment. This post delves into the process of developing and testing APIs on this https://127.0.0.1:1585 local setup, exploring best practices, tools, and strategies to achieve optimal results.
Setting Up the Development Environment
Localhost and Port Configuration
- Localhost (127.0.0.1): Represents the local machine, allowing developers to run and test applications in isolation from the external network.
- Port 1585: A non-standard port chosen to avoid conflicts with other services and to isolate the API for focused testing.
Step-by-Step Setup
1. Install Required Software:
Ensure you have the necessary software installed, such as a code editor (VS Code, Sublime Text), a local server (Node.js, Apache), and any API frameworks (Express.js, Flask, etc.).
2. Configure the Server
Set up your local server to listen on port 1585. For example, in an Express.js application:
javascript code
const express = require(‘express’);
const app = express();
const port = 1585;
app.listen(port, () => {
console.log(`API server running at https://127.0.0.1:${port}`);
});
3. Enable HTTPS:
To enable HTTPS, generate a self-signed SSL certificate using tools like OpenSSL. Then, configure your server to use these certificates.
javascript code
const https = require(‘https’);
const fs = require(‘fs’);
const options = {
key: fs.readFileSync(‘key.pem’),
cert: fs.readFileSync(‘cert.pem’)
};
https.createServer(options, app).listen(port, () => {
console.log(`Secure API server running at https://127.0.0.1:${port}`);
});
Developing APIs on https://127.0.0.1:1585
API Design Principles
- RESTful Design:
Follow REST principles for a structured, stateless API. Define clear endpoints, use standard HTTP methods (GET, POST, PUT, DELETE), and organize resources logically.
- Versioning:
Implement versioning (e.g., /api/v1/) to manage changes and updates to the API.
- Error Handling:
Ensure robust error handling to provide meaningful responses and HTTP status codes.
Example API Endpoints
- GET /api/v1/users: Retrieve a list of users.
- POST /api/v1/users: Create a new user.
- GET /api/v1/users/
: Retrieve a specific user by ID. - PUT /api/v1/users/
: Update user information by ID. - DELETE /api/v1/users/
: Delete a user by ID.
Testing APIs on https://127.0.0.1:1585
Importance of Testing
Testing ensures the API behaves as expected, handles edge cases gracefully, and maintains data integrity. It also helps identify and fix bugs early in the development process.
Types of Testing
- Unit Testing: This involves testing individual components or functions of the API. Frameworks such as Mocha, Jest, or PyTest are used.
- Integration Testing: Tests interactions between different parts of the API and external systems. Tools like Postman and Insomnia are useful for manual integration tests.
- End-to-End Testing: Simulates real-world scenarios to test the API’s overall functionality and performance. Tools like Cypress and Selenium can be used.
Setting Up a Test Suite
- Unit Tests:
Write unit tests for each function or module. For example, using Mocha and Chai for a Node.js API:
javascript code
const chai = require(‘chai’);
const chaiHttp = require(‘chai-http’);
const server = require(‘../server’); // Your Express app
const should = chai.should();
chai.use(chaiHttp);
describe(‘GET /api/v1/users’, () => {
it(‘should GET all the users’, (done) => {
chai.request(server)
.get(‘/api/v1/users’)
.end((err, res) => {
res.should.have.status(200);
res.body.should.be.a(‘array’);
done();
});
});
});
- Integration Tests:
Use Postman to create and run integration tests.
- Creating a Test Collection: Define a collection of requests corresponding to different API endpoints.
- Running Tests: Automate the execution of these tests and validate responses using Postman’s test scripts.
- End-to-End Tests:
Use Cypress for comprehensive testing.
javascript code
describe(‘User API Tests’, () => {
it(‘should create a new user and fetch it’, () => {
cy.request(‘POST’, ‘https://127.0.0.1:1585/api/v1/users’, { name: ‘John Doe’ })
.then((response) => {
expect(response.status).to.eq(201);
const userId = response.body.id;
return cy.request(‘GET’, `https://127.0.0.1:1585/api/v1/users/${userId}`);
})
.then((response) => {
expect(response.status).to.eq(200);
expect(response.body.name).to.eq(‘John Doe’);
});
});
});
Best Practices for Developing and Testing APIs
Documentation
- API Documentation: Use tools like Swagger or Postman to generate interactive API documentation.
- Code Comments: Comment your code to explain logic and functionality, making it easier for others to understand and maintain.
Continuous Integration/Continuous Deployment (CI/CD)
- Automated Testing: Integrate automated testing into your CI/CD pipeline to run tests on every commit or pull request.
- Deployment: To automate deployment processes, use CI/CD tools like Jenkins, Travis CI, or GitHub Actions.
Security Measures
- Input Validation: Validate all inputs to prevent SQL injection, XSS, and other security vulnerabilities.
- Authentication and Authorization: To secure your API, implement robust authentication (e.g., OAuth, JWT) and authorization mechanisms.
Monitoring and Maintenance
Performance Monitoring
- Tools: Monitoring tools like New Relic, Datadog, or Prometheus can be used to track API performance and health.
- Metrics: Monitor critical metrics such as response times, error rates, and throughput to identify and resolve performance issues.
Regular Updates and Patches
- Dependency Management: Keep your dependencies up to date to ensure security and compatibility.
- Code Refactoring: Regularly review and refactor code to improve maintainability and performance.
Conclusion
Developing and testing APIs on https://127.0.0.1:1585 provides a secure and controlled environment for ensuring the quality and reliability of your applications. By following best practices for development, implementing comprehensive testing strategies, and leveraging modern tools and frameworks, developers can create robust APIs that meet user needs and withstand real-world usage. Continuous monitoring, documentation, and maintenance ensure that the APIs remain secure, efficient, and up-to-date, providing a solid foundation for any software application.