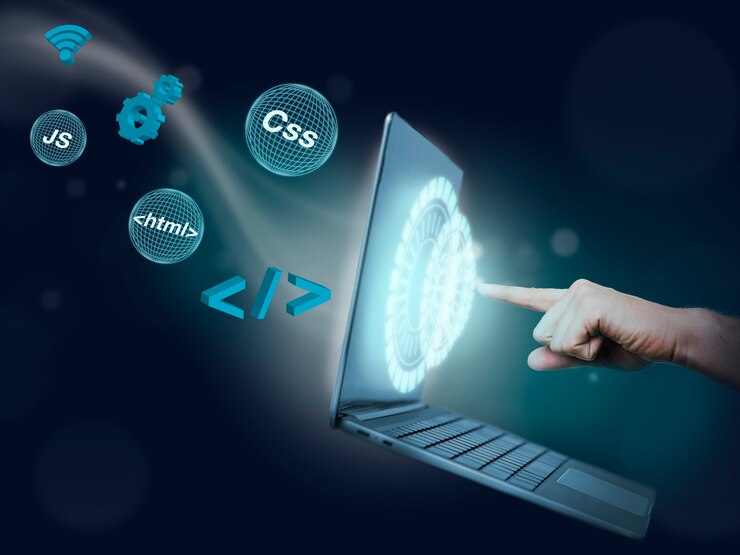
Web scraping is the process of extracting data from websites. This technique is used to collect valuable information like prices, product details, news articles, or even social media content. If you’re a beginner, starting a web scraping project may seem overwhelming, but with the right approach, you can build your own project easily. In this guide, we’ll walk through the steps to create a simple web scraping project.
What is a Web Scraping Project?
A web scraping project involves writing a program that can access web pages, extract specific information from them, and save it in a structured format like CSV, JSON, or a database. Whether you want to gather data for research, business analysis, or personal use, web scraping is a powerful tool to automate the data collection process.
Why Should You Build a Web Scraping Project?
There are several reasons why building a web scraping project can be valuable:
- Automate Data Collection: Save time by automatically collecting data instead of doing it manually.
- Access to Structured Data: Transform unstructured data from websites into a clean, usable format.
- Real-World Application: Build skills that can be applied to real-world tasks like data analysis, market research, and more.
Tools You Need for Your Web Scraping Project
Before you start your web scraping project, you’ll need some basic tools. The most commonly used tools for web scraping are:
- Python: Python is a popular programming language for web scraping because of its simplicity and a wide range of libraries.
- Libraries:
- BeautifulSoup: A Python library for parsing HTML and XML documents.
- Requests: A simple library to send HTTP requests and get web pages.
- Pandas: A library for handling and analyzing data, which helps in saving the scraped data in a structured format.
- Text Editor or IDE: Use a text editor like Visual Studio Code or PyCharm to write your code.
Step-by-Step Guide to Build a Web Scraping Project
Step 1: Install the Required Libraries
First, you need to install the necessary libraries for your web scraping project. Open your terminal or command prompt and run the following commands:
pip install requests
pip install beautifulsoup4
pip install pandas
This will install the requests library for making HTTP requests, BeautifulSoup for parsing HTML, and Pandas for saving the scraped data.
Step 2: Choose a Website to Scrape
Next, select a website from which you want to scrape data. For beginners, it’s best to start with a simple, static website, as dynamic websites (like those with JavaScript) can be more complicated to scrape. Websites like quotes.toscrape.com or books.toscrape.com are perfect for beginners because they are designed for practice.
Make sure you review the website’s robots.txt
file to ensure you’re allowed to scrape it.
Step 3: Send a Request to the Website
To scrape data from a website, you first need to send an HTTP request to the website’s server. This can be done using the requests library. Here’s how you can send a GET request to a website:
import requests
url = "http://quotes.toscrape.com/"
response = requests.get(url)
# Check if the request was successful
if response.status_code == 200:
print("Successfully fetched the webpage!")
else:
print("Failed to fetch the webpage!")
Step 4: Parse the HTML Content
Once you’ve received the HTML content from the website, you need to parse it and extract the information you need. This is where BeautifulSoup comes in. BeautifulSoup allows you to easily navigate the HTML tree and find the specific elements you’re interested in.
Here’s an example of how to parse the HTML:
from bs4 import BeautifulSoup
# Parse the HTML content
soup = BeautifulSoup(response.text, "html.parser")
# Find all quotes on the page
quotes = soup.find_all("span", class_="text")
# Print the quotes
for quote in quotes:
print(quote.get_text())
This code extracts all the text inside the <span>
tags with the class text
, which contain the quotes on the webpage.
Step 5: Save the Scraped Data
Now that you’ve extracted the data you need, you can save it into a structured format. A common format is a CSV file, which can easily be opened in Excel or analyzed in Python using Pandas.
Here’s how to save the scraped data into a CSV file:
import pandas as pd
# Create a list to hold the quotes
quotes_list = []
# Extract quotes and authors
for quote in quotes:
quotes_list.append({"quote": quote.get_text()})
# Convert the list to a DataFrame and save it as CSV
df = pd.DataFrame(quotes_list)
df.to_csv("quotes.csv", index=False)
print("Data saved to quotes.csv!")
This will save the scraped quotes into a CSV file called quotes.csv
.
Step 6: Run the Web Scraping Project
After setting everything up, run your script. It will send a request to the website, extract the data, and save it to a CSV file.
python scrape_quotes.py
Best Practices for Your Web Scraping Project
While scraping, it’s important to follow some best practices to avoid potential issues:
- Respect the Website’s Terms of Service: Always check the website’s
robots.txt
file to ensure you are allowed to scrape. - Don’t Overload the Server: Use time delays (e.g.,
time.sleep()
) between requests to avoid overwhelming the server. - Handle Errors Gracefully: Add error handling in your code to deal with issues like connection errors, missing elements, or empty pages.
- Stay Organized: Keep your project organized with proper folder structures and clean, readable code.
Conclusion
Building a web scraping project can be a fun and rewarding experience. By following the simple steps outlined in this guide, you can start scraping websites and collecting useful data in no time. From sending requests to parsing HTML and saving data, each step is an essential part of building your first web scraping project.
With more experience, you can work on more complex scraping tasks, handle dynamic websites, and even scale up your project for larger data collections. Always remember to follow best practices, be respectful of website owners, and refine your skills as you go. Happy scraping!