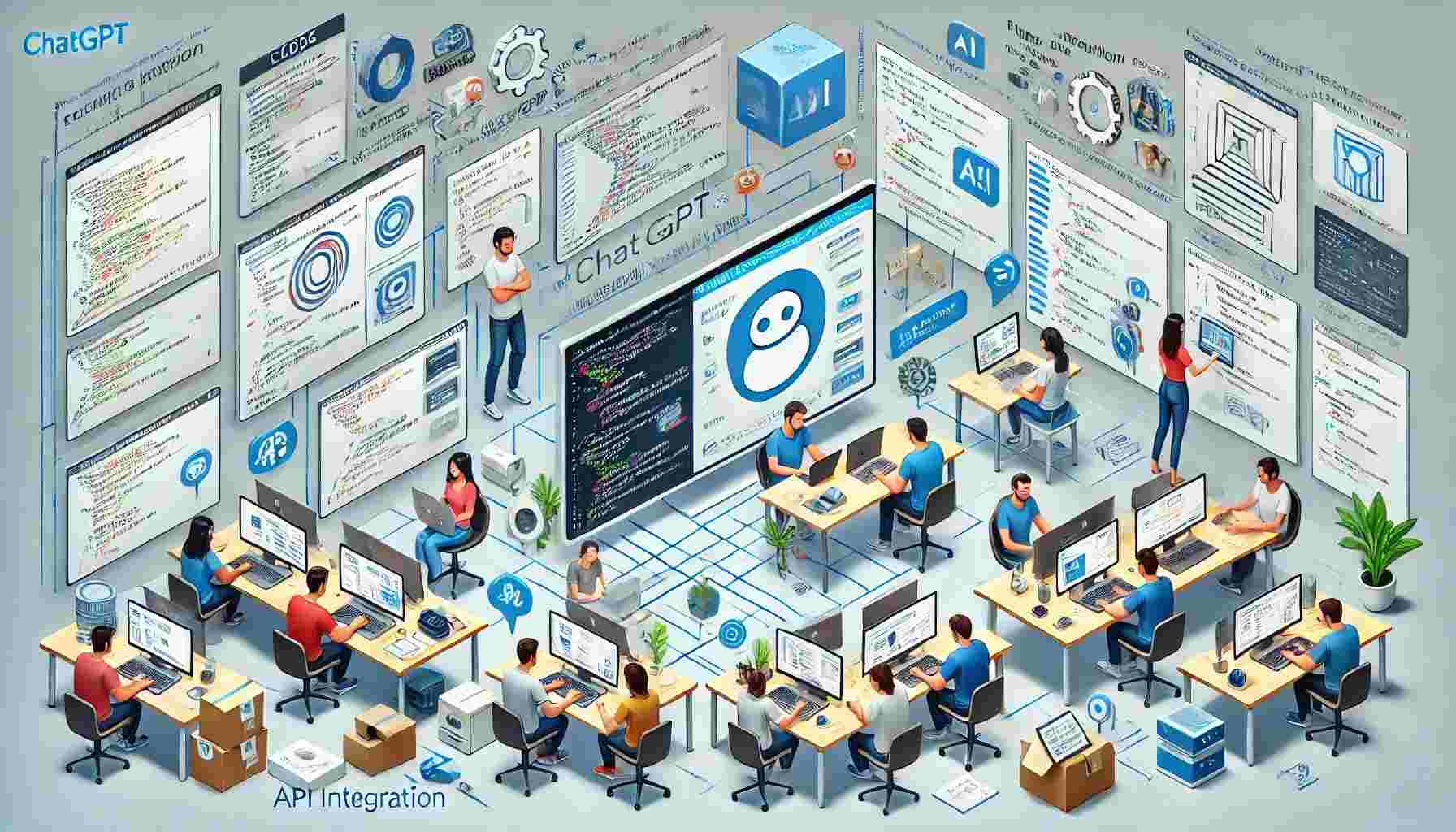
Adding ChatGPT to mobile apps can improve user experience by offering advanced conversation. OpenAI developed ChatGPT-Powered Mobile Apps, a powerful language model that can understand and generate human-like text. This makes it ideal for chatbots, virtual assistants, and other conversational interfaces. This guide explores the steps and best practices for building ChatGPT-powered mobile apps.
1. Understanding ChatGPT and Its Capabilities
What is ChatGPT?
ChatGPT is a variant of the GPT model designed to generate clear and relevant text. It can understand natural language, engage in dialogue, answer questions, and perform text-based tasks.
Capabilities and Use Cases
- Conversational Interfaces: Chatbots and virtual assistants for customer support, personal assistance, and more.
- Content Generation: Creating articles, summaries, and other text content.
- Language Translation and Interpretation: Real-time language translation and interpretation services.
- Education and Tutoring: Providing explanations, tutoring, and educational content.
2. Setting Up the Development Environment
Choosing the Technology Stack
- Mobile Frameworks: Choose a mobile framework such as React Native, Flutter, or native development with Swift (iOS) and Kotlin (Android).
- Backend Services: Use backend services to manage API calls and handle the integration with OpenAI’s API.
- APIs and SDKs: Utilize OpenAI’s API to access ChatGPT-Powered Mobile Apps’s capabilities.
Development Tools
- IDE: Integrated Development Environment such as Visual Studio Code, Android Studio, or Xcode.
- Version Control: Git for version control and collaboration.
- Testing Tools: Tools like Jest, Detox, or Espresso for testing the app.
3. Integrating ChatGPT into Your Mobile App
Creating an OpenAI Account and API Key
- Sign Up: Create an account on the OpenAI platform.
- API Key: Obtain your API key from the OpenAI dashboard. This key will be used to authenticate your app’s requests to the ChatGPT API.
Setting Up the Backend
- Server Setup: Set up a server using Node.js, Python, or any other backend technology.
- API Requests: Implement the logic to handle API requests to the OpenAI endpoint. Use the API key to authenticate these requests.
- Response Handling: Process and handle the responses from ChatGPT to ensure they are correctly formatted and contextually relevant.
Example Code (Node.js):
javascript
Copy code
const express = require(‘express’);
const axios = require(‘axios’);
const app = express();
const port = 3000;
app.use(express.json());
const OPENAI_API_KEY = ‘your-api-key’;
app.post(‘/chat’, async (req, res) => {
const userMessage = req.body.message;
try {
const response = await Axios.post(‘https://api.openai.com/v1/engines/davinci-codex/completions’, {
prompt: user message,
max_tokens: 150,
temperature: 0.7
}, {
headers: {
‘Authorization’: `Bearer ${OPENAI_API_KEY}`
}
});
res.json({ message: response.data.choices[0].text });
} catch (error) {
console.error(‘Error:’, error);
res.status(500).send(‘Internal Server Error’);
}
});
app.listen(port, () => {
console.log(`Server is running on http://localhost:${port}`);
});
Integrating with the Mobile App
- API Call: Implement the logic to make HTTP requests from your mobile app to the backend.
- UI Design: Design the chat interface using your chosen mobile framework. Ensure the UI is intuitive and user-friendly.
- State Management: Manage the conversation’s state to maintain the context between the user’s messages and ChatGPT’s responses.
Example Code (React Native):
javascript
Copy code
import React, { useState } from ‘react’;
import { View, Text, TextInput, Button, FlatList } from ‘react-native’;
import axios from ‘axios’;
const ChatScreen = () => {
const [messages, setMessages] = useState([]);
const [input, setInput] = useState(”);
const sendMessage = async () => {
if (input.trim() === ”) return;
const userMessage = { type: ‘user’, text: input };
setMessages([…messages, userMessage]);
try {
const response = await axios.post(‘http://localhost:3000/chat’, { message: input });
const botMessage = { type: ‘bot’, text: response.data.message };
setMessages([…messages, userMessage, botMessage]);
} catch (error) {
console.error(‘Error:’, error);
}
setInput(”);
};
return (
<View style={{ flex: 1, padding: 20 }}>
<FlatList
data={messages}
keyExtractor={(item, index) => index.toString()}
renderItem={({ item }) => (
<View style={{ padding: 10, backgroundColor: item.type === ‘user’ ? ‘#DCF8C6’ : ‘#ECECEC’, alignSelf: item.type === ‘user’ ? ‘flex-end’ : ‘flex-start’, border-radius: 5, marginVertical: 5 }}>
<Text>{item.text}</Text>
</View>
)}
/>
<TextInput
value={input}
onChangeText={setInput}
placeholder=”Type a message”
style={{ borderWidth: 1, borderColor: ‘#CCCCCC’, padding: 10, borderRadius: 5, marginVertical: 10 }}
/>
<Button title=”Send” onPress={sendMessage} />
</View>
);
};
export default ChatScreen;
4. Enhancing the Chat Experience
Natural Language Understanding
Add NLU to enhance the chatbot. It will understand and process user input, handle user intents, recognize entities, and keep context during the conversation.
Context Management
Managing the conversation’s context is crucial. It helps in giving clear and relevant responses. Use session variables or state management solutions to track the conversation’s context.
Personalization
Personalize the chatbot experience by using user data and preferences. This can involve tailoring responses based on user history, preferences, and past interactions.
Error Handling
Implement robust error handling to manage unexpected inputs or issues with the ChatGPT API. Provide informative and friendly messages to guide users when errors occur.
5. Testing and Deployment
Testing
- Unit Testing: Test individual components and functions to ensure they work correctly.
- Integration Testing: Test the mobile app, backend, and ChatGPT API integration to ensure seamless communication.
- User Testing: Conduct user testing to gather feedback on the chatbot’s performance and user experience.
Deployment
- Backend Deployment: Deploy the backend server to a cloud platform like AWS, Google Cloud, or Heroku.
- Mobile App Deployment: Publish the mobile app to app stores (Apple App Store for iOS and Google Play Store for Android).
- Monitoring and Maintenance: Monitor the performance of the chatbot and the backend server. Regularly update and maintain the system to ensure optimal performance and security.
6. Case Studies and Examples
Case Study 1: E-commerce Chatbot
An e-commerce company implemented a ChatGPT-powered chatbot. The chatbot assists customers with product inquiries, order tracking, and support requests. The chatbot improved customer engagement and reduced response times, increasing customer satisfaction and sales.
Case Study 2: Health Advisory App
A health advisory app is integrated with ChatGPT. It gives users personalized health advice and information. The chatbot understands normal language and responds well, improving the app’s usability and user trust.
Case Study 3: Educational Tutor
An educational platform used ChatGPT to develop a virtual tutor. The tutor helps students with their studies. The chatbot provided explanations, answered questions, and offered personalized study recommendations, improving the learning experience for students.
Conclusion
Making ChatGPT-powered mobile apps involves understanding ChatGPT’s abilities. You also set up a development environment and integrate the API. Then, you optimize the user experience. Finally, you ensure robust testing and deployment. Developers can use ChatGPT’s advanced conversation abilities. They can create engaging, responsive, and smart mobile apps. These apps improve how users interact and their satisfaction. Conversational AI is evolving. Adding ChatGPT to mobile apps will become more valuable. It will be valuable for developers who want to create innovative, user-focused apps.