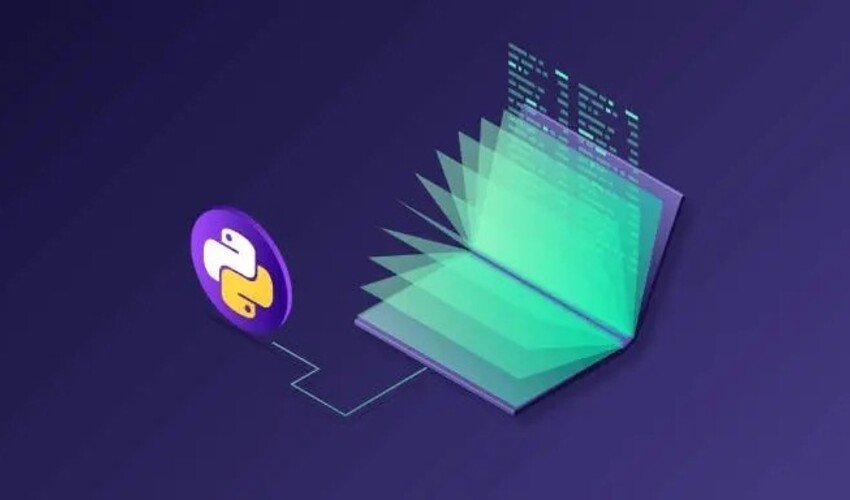
In Python, the sleep()
function allows you to pause the program for a specified amount of time. This can be useful if you want to delay the execution of a program or create a wait time between certain actions. The sleep()
function is part of the time
module, which means you first need to import it before using it.
How to Use Sleep in Python
To use the sleep()
function, follow these simple steps:
- Import the Time Module First, you need to import the
time
module into your program. This module contains thesleep()
function.import time
- Use the Sleep Function After importing the module, you can use the
sleep()
function. The function takes one argument: the number of seconds you want the program to wait.time.sleep(3)
In the example above, the program will pause for 3 seconds before moving to the next line of code. - Example Code: Here’s a simple program that uses
sleep()
to create a pause between printing messages:import time print("Hello!") time.sleep(2) # Pause for 2 seconds print("How are you?") time.sleep(1) # Pause for 1 second print("Goodbye!")
In this example, the program will print “Hello!”, wait for 2 seconds, then print “How are you?”, wait for 1 second, and finally print “Goodbye!”.
Why Use Sleep in Python?
The sleep()
function is helpful in many situations, such as:
- Delaying code execution: If you want to slow down or pause your program for a specific amount of time.
- Simulating wait time: For example, when you are simulating the process of checking data or making requests.
- Creating animations or games: Sleep can be used to make objects or characters move at a slower pace.
Important Notes:
- The argument passed to
sleep()
is in seconds, sotime.sleep(2)
means the program will pause for 2 seconds. - You can also use decimal values for more precise sleep times, like
time.sleep(0.5)
for a half-second pause. sleep()
is often used in games, automation scripts, or any program where you want to introduce delays.
FAQs
1. What happens if I don’t import the time module?
If you forget to import the time
module and try to use time.sleep()
, you’ll get an error. Make sure to include import time
at the beginning of your code.
2. Can I use decimal numbers with sleep()?
Yes, you can. For example, time.sleep(0.5)
will pause the program for half a second.
3. How can I stop a program from sleeping?
If you want to stop or interrupt the sleep process, you can use the keyboard shortcut Ctrl + C
in most terminal environments, which will stop the program.
4. Is there a way to speed up or shorten the time in sleep()
?
Yes, you can change the number of seconds in sleep()
. For example, using time.sleep(0.1)
will make the program pause for 0.1 seconds.
5. Can I use sleep in a loop?
Yes, you can use sleep()
inside a loop to create pauses between iterations. For example:
import time
for i in range(5):
print(i)
time.sleep(1)
This will print numbers from 0 to 4, pausing for 1 second between each number.
Also read about Python Spark