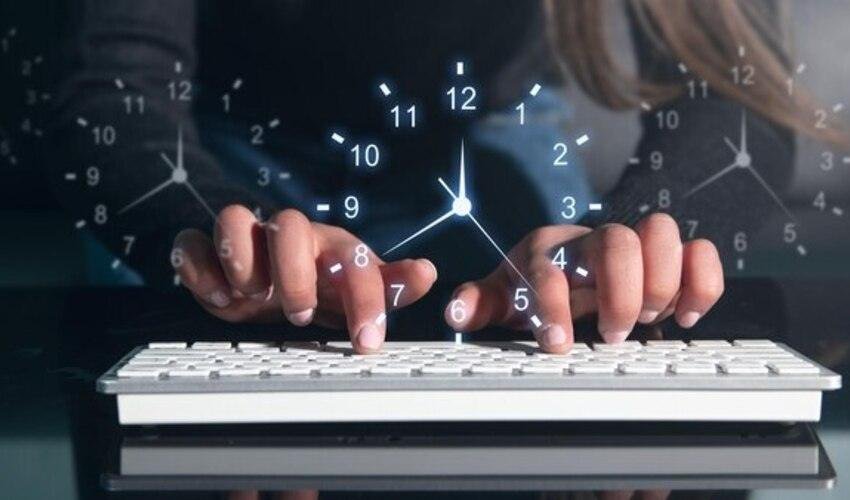
Selection Sort is a simple sorting algorithm. It works by finding the smallest element in the list. The smallest element is then swapped with the first unsorted element. This process repeats until the entire list is sorted. In this article, we will discuss the Selection Sort Time Complexity in detail.
How Selection Sort Works
Selection Sort divides the list into two parts: sorted and unsorted. It picks the smallest element from the unsorted part. The smallest element is then placed in the correct position. This process continues until all elements are sorted. The number of comparisons remains constant for a given list size.
Understanding Big O Notation
Big O Notation describes how an algorithm’s running time grows. It helps analyze an algorithm’s efficiency. The notation provides worst-case, best-case, and average-case complexities. Understanding Selection Sort Time Complexity helps in deciding when to use it.
Worst-Case Time Complexity
The worst case occurs when the list is sorted in reverse order. The algorithm must compare every element. The number of comparisons for Selection Sort Time Complexity is calculated as follows:
- First pass: comparisons
- Second pass: comparisons
- Third pass: comparisons
- …
- Last pass: 1 comparison
Using Big O notation, this simplifies to O(n²). The worst-case Selection Sort Time Complexity is quadratic.
Best-Case Time Complexity
The best case occurs when the list is already sorted. However, Selection Sort still makes comparisons. The number of comparisons remains the same. Thus, even in the best case, Selection Sort Time Complexity is O(n²).
Average-Case Time Complexity
The average case assumes a random order of elements. The number of comparisons remains approximately the same. Swaps may be different, but comparisons dominate. So, the Selection Sort Time Complexity for the average case is also O(n²).
Space Complexity of Selection Sort
Selection Sort does not require extra space. It sorts the list in place. The space complexity is O(1) because it only uses a few extra variables.
When to Use Selection Sort
Selection Sort is useful for small lists. It performs well when swaps are expensive. It is not efficient for large datasets because of its O(n²) time complexity.
Comparison with Other Sorting Algorithms
- Bubble Sort: Similar O(n²) complexity but performs more swaps.
- Insertion Sort: Faster on nearly sorted data.
- Merge Sort: More efficient with O(n log n) complexity.
- Quick Sort: Generally faster but uses extra space.
Conclusion
Selection Sort is easy to understand and implement. However, its Selection Sort Time Complexity makes it slow for large lists. It is useful when minimizing swaps is important. Understanding Selection Sort Time Complexity helps in choosing the right sorting algorithm.