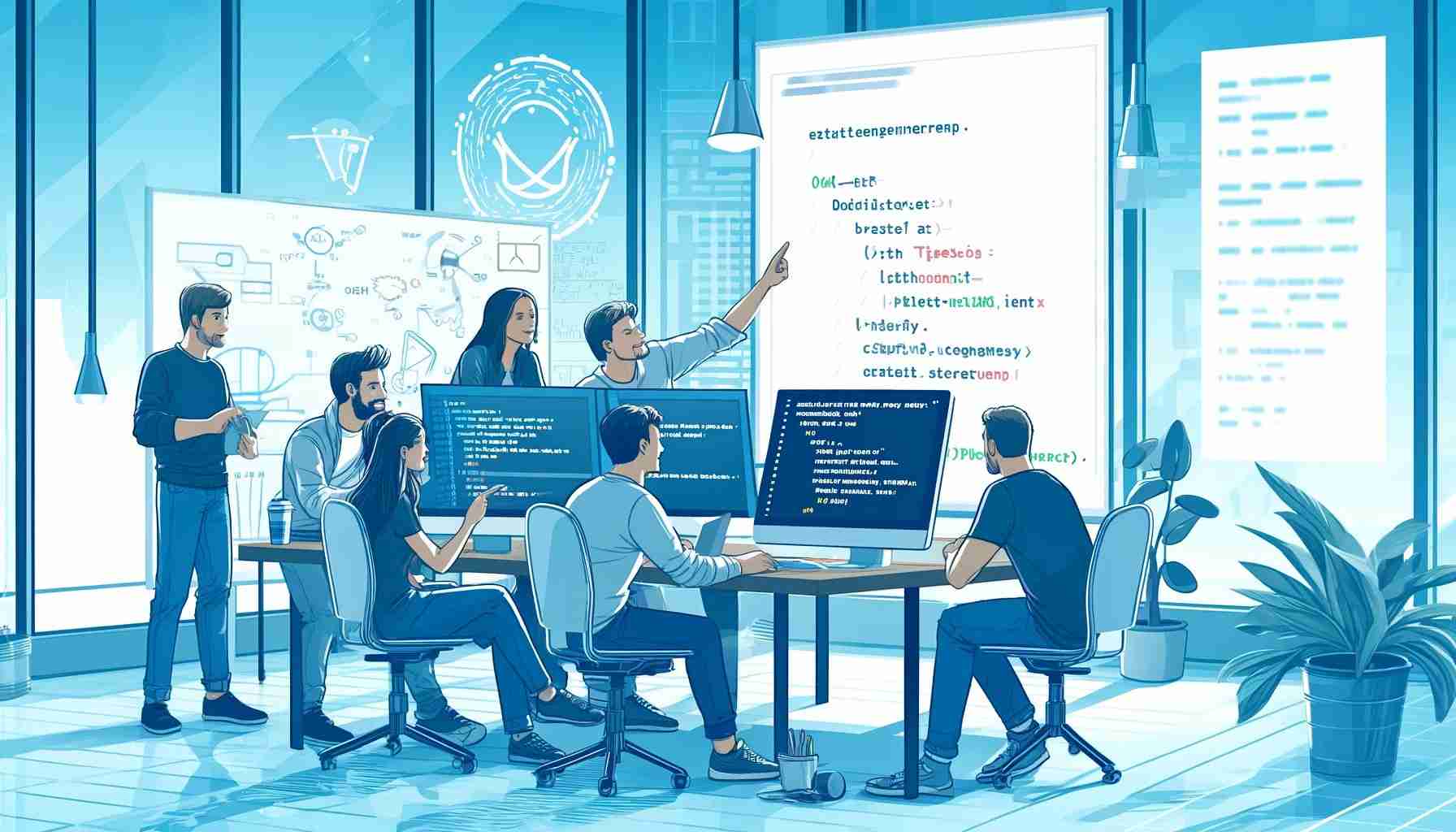
The combination of Zustand and TypeScript has become popular with developers because of its numerous advantages in web development. Zustand and TypeScript are powerful JavaScript supersets that add static typing to the language, making it easy and fast to manage the global state. By combining them, Zustand and TypeScript create a strong and efficient environment for the development of modern web applications.
Zustand
Zustand is a lightweight state management library that provides a simple and elegant way to manage an application’s state.
.Why do you use TypeScript in Zustand?
In combination with TypeScript, developers can use robust typing and type checking to detect errors at compile time instead of during the run. This creates a more robust, reliable, optimized code with fewer bugs and a smoother development process. By checking static types, TypeScript brings strong typing to JavaScript, improving the quality and reliability of the code. TypeScript will help to ensure more secure and predictable application state management when used in Zustand. It makes it easier to refactor, debug, and test the state by ensuring that the state and its transitions are well-defined and conform to the expected types.
Core Concepts of Zustand
- Store: The central concept in Zustand is the “store.” A store in Zustand is where your application’s state lives. It’s created using the
create
function from Zustand, and it can contain any valid JavaScript value. - Hooks: Zustand uses custom hooks (e.g.,
useStore
) to let React components subscribe to store updates. This approach aligns well with modern React development, embracing hooks for state management. - Immutability: Although Zustand doesn’t enforce immutability, it’s recommended to treat the state as immutable to prevent bugs. Zustand stores can be configured to work with popular immutability helpers or libraries if needed.
Setting Up Zustand with TypeScript
To start using Zustand with TypeScript in a React project, you need to define the types for your store’s state and actions. Here’s a basic setup:
typescriptCopy codeimport create from 'zustand'
// Define the state's shape with TypeScript interfaces or types
interface CounterState {
count: number;
increment: () => void;
decrement: () => void;
}
// Create the store using the `create` function from Zustand
const useStore = create<CounterState>((set) => ({
count: 0,
increment: () => set(state => ({ count: state.count + 1 })),
decrement: () => set(state => ({ count: state.count - 1 })),
}));
// In a React component
function Counter() {
const { count, increment, decrement } = useStore();
return (
<div>
<p>{count}</p>
<button onClick={increment}>+</button>
<button onClick={decrement}>-</button>
</div>
);
}
This simple example shows how to set up a counter store with increment and decrement actions. The state and actions are strongly typed, ensuring they are used correctly across your application.
Benefits of Using Zustand with TypeScript
Some benefits are listed here:
Improved developer experience
Improved developer experience is one of the most important benefits associated with Zustand and TypeScript. TypeScript’s robust type system allows developers to easily define and enforce strict data types for state management, improving the quality and maintainability of code. Additionally, Zustand’s simple API and intuitive syntax make it accessible and user-friendly, even for those new to programming.
High Optimization
Zustand is optimized and efficient, making it ideal for managing complex state logic while maintaining performance. By utilizing TypeScript’s static typing, developers can write more reliable code that runs faster and uses fewer resources.
More rapid Scaling
The combination of Zustand and TypeScript allows for more rapid scaling when it comes to complex applications. Zustand’s unique approach to global governance will enable developers to effortlessly scale their applications without the constraints of traditional state management systems. TypeScript’s robust type security further simplifies code maintenance and expansion, ensuring a smooth transition as your project grows in complexity.
Enhance code maintainability and readability
Zustand’s use of TypeScript can improve code retention and readability. With TypeScript type annotations, developers can quickly understand the structure of a state and its interactions, making it easier for them to reason about code and make changes without fear of introducing unexpected bugs. Zustand’s compact, lightweight nature also makes it easy to keep the codebase current and updated over time.
Conclusion
Finally, it is clear that Zustand with TypeScript improves the developer’s experience, enhances performance, and increases scale and code stability. Leveraging the strengths of these two tools allows developers to create robust, flexible, and easily maintained web apps. The integration of Zustand with TypeScript in your workflow can greatly enhance project quality and productivity, whether you’re an experienced developer or a newcomer.
Overall, the combination of Zustand with TypeScript provides an effective and flexible solution for state management in modern web development. With the use of both tools, developers can develop effective, scalable, and maintenanceable applications that meet the demands of today’s rapidly evolving development environment. With their combination, you can help raise your code to new levels of quality and performance while working on small projects or large corporate applications.