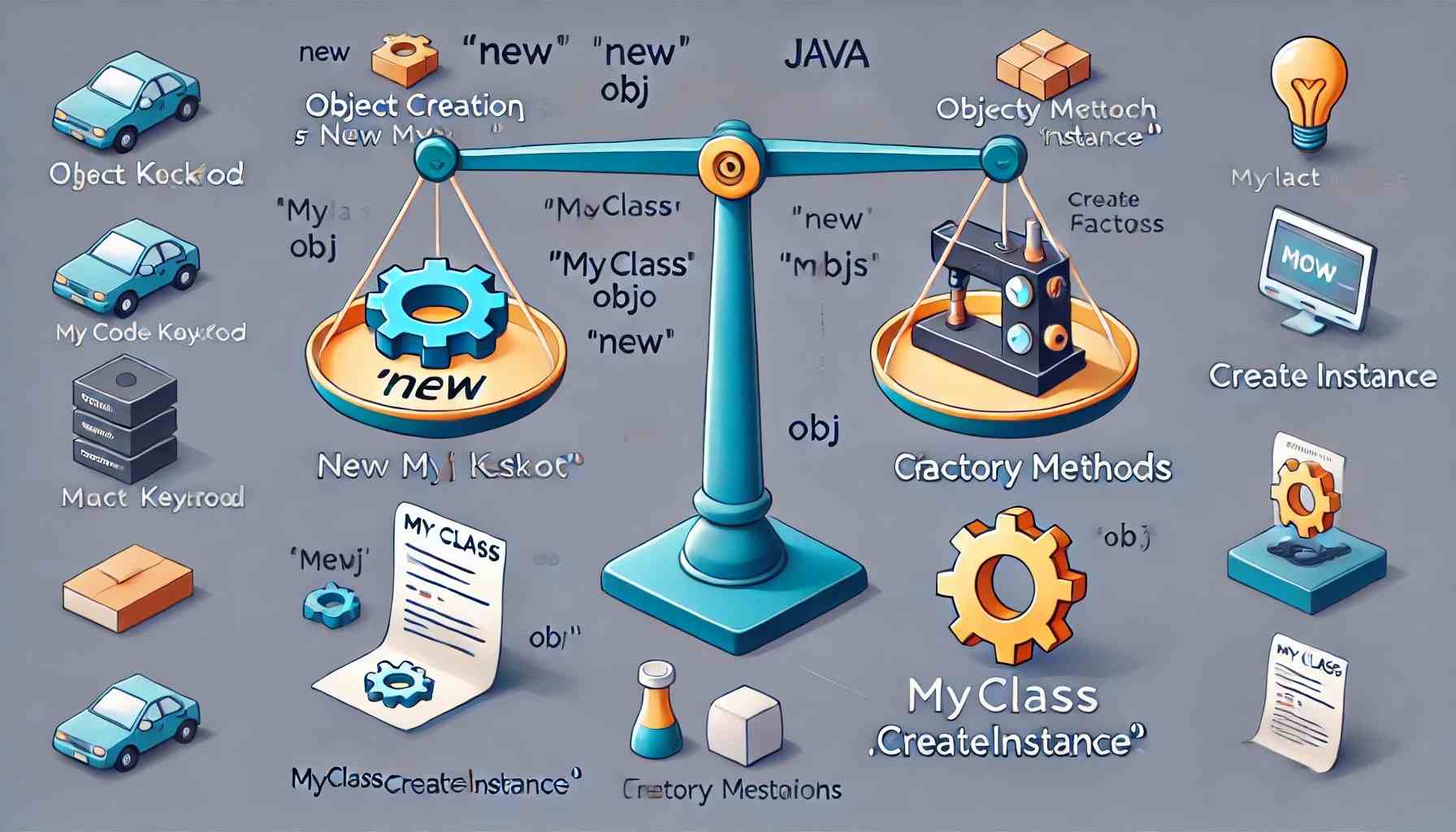
Last updated on August 23rd, 2024 at 01:04 pm
In Java, instantiation of objects is an important process of handling the language, and the two most basic techniques include the new keyword and factory methods. Each of the communities can be effective, and the choice between them depends on certain design factors. In this article, I will discuss various features of the two techniques, compare and contrast them, and answer the question when one should use one or the other.
Objects creation with the help of the ‘new
’ keyword
The easiest method of generating an object in Java is by the use of new
operator. This method is one of the first to be introduced to learners in Java programming classes and is common in basic applications.
Example
MyClass obj = new MyClass();
In this approach:
- A new object of MyClass is developing.
- All dynamic objects are stored in the heap, and therefore memory is provided to the object from there.
- The constructor of MyClass is called and the object of MyClass is created.
This method therefore directly links the code to the class of the object that is being instantiated. Despite the fact that it is elementary and straightforward, it has some drawbacks, which are pronounced in more complex programs.
Advantages of Using the new
Keyword:
Simplicity: Since it is quite easy to understand and implement, it can be adopted for small or simple instances.
Direct control: The objects in this scenario are directly created and possibly controlled by the developer in how a certain constructor is chosen.
Disadvantages of Using the new
Keyword:
Tight Coupling: Extensive use of new is synchronization with the class in the process of instantiation. When the class evolves (for example when the constructor or the type of the object changes), all the references to new has to be revised.
Limited flexibility: It does not support object creation where the setup process or if they are reusable may be a bit complicated. This method has some disadvantage that it is not easy to separate the creational logic with the implementation.
Object Creation Using Factory Methods
Factory methods are a way of creating objects that is offered by object oriented programming and it differs from the use of the “new” keyword in parts of code. It makes the object creation process stronger and more flexible because factory method contains the creation logic itself.
Example
MyClass obj = MyClass.createInstance();
In this example, though the object is not directly created using the new keyword but through a factory method (createInstance()). The factory method might also include some logic before the instantiation, for example set the default values, meeting some conditions, or even it may return another subclass or an object of a different implementation of an interface.
Advantages of Factory Methods:
Decoupling: The applicant who uses the factory method should not have details of the object creation as required by the program. It just has to throw a request to the factory and gets back an object.
Enhanced Flexibility: Factory methods polymorphic, can return object of a different class, which can be useful when dealing with an interface or an abstract class.
Control Over Creation: Yes, IT specialists can set up rules that would force creation of objects. For example, you can return singleton instances, use an existing object (kind of object pooling), or return different objects of different types based on some parameters or conditions.
Improved Testability: Another thing which makes it easy to mock or replace objects in the course of unit tests is that their creation is also centralized in a factory.
Disadvantages of Factory Methods:
Complexity: Factory methods bring a level of indirection that some developers will find unpleasant if they are new to the language.
Overhead: Sometimes, the employment of a factory method allows for extra load which may mean that the method gets complicated.
When to Use “new
” Keyword
The new
keyword is useful when you have small, uncomplicated application or when you are creating an instance of a particular class when the complexity is not high. It works best when: The class constructor is easy and no further preparations or tests are needed for creating the class.
You don’t have to separate the creation of objects from the objects themselves by means of a function pointer.
The code was designed not to have to require much if any maintenance or expansion in the future.
For instance, the new operator fits especially well where you need to use small and portable prototypes, or where developing code that will immediately be discarded.
When to Use Factory methods
Factory methods should be used as soon as there is a need to create object and when flexibility of the application is desired.
Factory methods are best suitable for large scale complex applications where there is high level of flexibility and decoupling is required while doing maintenance.
They are particularly beneficial when:
- You have to ensure particular rules of object creation or Object reuse effectively.
- Your application involves interfaces, abstract classes, or polymorphism, and the exact object type may vary.
- You want to follow design patterns like Singleton, Abstract Factory, or Builder.
- You anticipate frequent changes in the object creation logic, as you can modify the factory without affecting other parts of the code.
Comparison of the new
keyword versus Factory Methods in Java
Feature | new Keyword | Factory Methods |
---|---|---|
Purpose | Used to create a new instance of a class. | Used to control object creation and provide flexibility. |
Object Creation | Direct instantiation of a class using new . | Object creation is delegated to a method. Can return existing instances or new ones. |
Syntax | ClassName obj = new ClassName(); | ClassName obj = ClassName.factoryMethod(); |
Flexibility | Less flexible, always creates a new instance. | More flexible, can return cached, singleton, or subclass objects. |
Performance | Always allocates memory for a new object. | Can optimize performance by reusing objects. |
Return Type | Always returns an instance of the class being created. | Can return an instance of the same class, a subclass, or even a different type. |
Object Pooling | Not possible, always creates a new object. | Can implement object pooling, returning pre-existing objects when necessary. |
Encapsulation | Less encapsulated, as the constructor is directly called. | More encapsulated, hides the instantiation logic from the user. |
Extensibility | Harder to change the object creation process without modifying the code that uses new . | Easier to extend and modify object creation logic without changing client code. |
Constructor Visibility | Requires the constructor to be accessible (public). | The constructor can be private, allowing the factory method to control access. |
Singleton Pattern | Difficult to enforce singleton pattern without additional logic. | Easily supports singleton pattern by returning the same instance every time. |
Abstract Classes / Interfaces | Cannot instantiate abstract classes or interfaces. | Can return instances of abstract classes, interfaces, or their implementations. |
Examples | Person p = new Person(); | Person p = Person.createPerson(); |
Conclusion
As became apparent from the previous sections, although the new
keyword is a straightforward method to generate the objects in Java, it is not as versatile as is required in large systems. Factory methods are more preferable as they allow to control object creation to a greater extent and manage it, as well as to ensure decoupling and better manage code maintenance. While choosing which approach of the two is desirable to be applied, some aspects to be considered by the developers include; The level of complexity of the application The flexibility required The issue of maintainability in the future. For small, clearcut tasks ‘New’ may be sufficient while for large growing applications, ‘Factory methods’ are usually the best.
FAQ’s
What is the phenomenal variation between two approaches for creating objects: new
keyword vs. factory methods?
“new” keyword immediately calls the class constructor, being the factory methods to hide the creation process thus enabling more of object creation options. Factory methods can also returns different types of objects, preconditions and instance reuse.
When would this be preferable to use instead of a factory method?
The new keyword is a perfect fit for small applications when complexity has to be avoided and such things as flexible object creation are not necessary. However, when the object creation process is simple and is not expected to be altered in the near future, new is a great way to go.
Why Indeed are Factory methods employed so frequently particularly in larger applications?
Important, factory methods disassociation the creation of objects from the using class what increase the maintainability and flexibility. They permit you to make complex objects, object stacking and better utilizing of design patterns as the Singleton or Factory making them more relevant for vast, scalable projects.
Can I use both new
keyword and factory methods in the same application?
Yes, it’s common to use both approaches in different parts of an application. Simple objects that don’t require any special creation logic can be instantiated with new
, while more complex objects or objects that require decoupling can be created using factory methods.