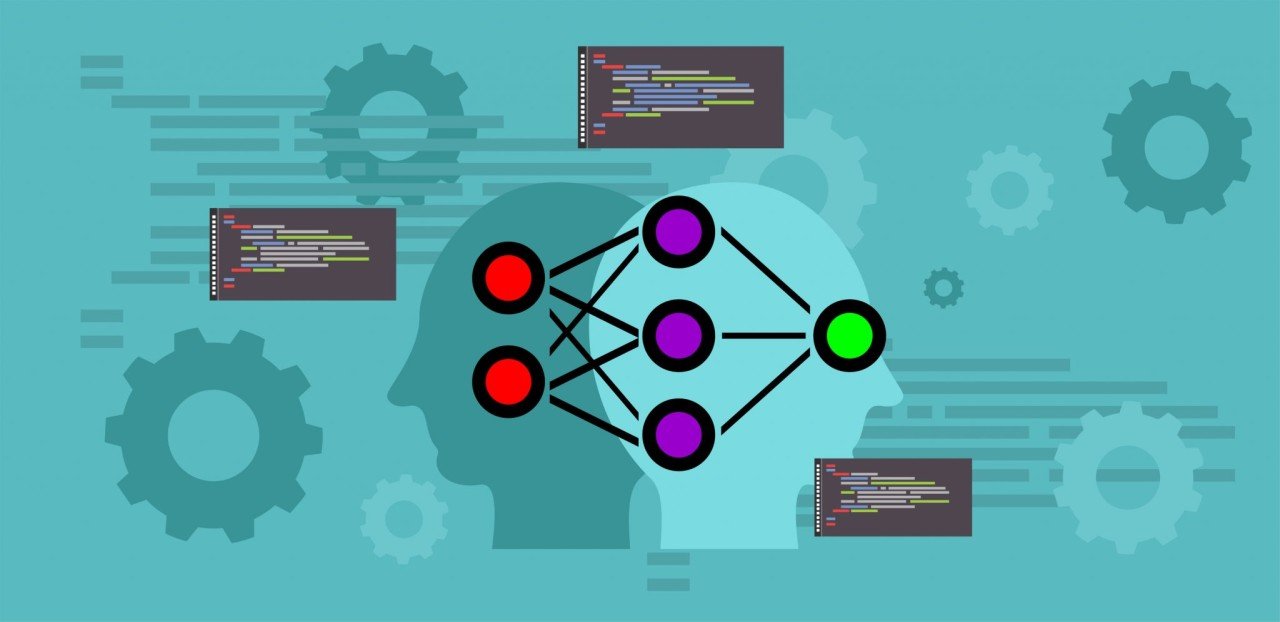
Sorting is one of the most fundamental tasks in computer science, and understanding how sorting algorithms work can help programmers choose the best method for organizing data. Among the many sorting algorithms, Swap Sort is one of the simplest. In this article, we’ll take a deep dive into swap sort, how it works, and when you might want to use it.
What is Swap Sort?
Swap Sort is a simple sorting algorithm based on the idea of repeatedly swapping adjacent elements that are out of order until the entire list is sorted. It’s similar in behavior to Bubble Sort but is often less efficient. While Swap Sort is intuitive and easy to implement, it’s not commonly used in practice due to its inefficiency compared to more advanced sorting algorithms like Quick-Sort or Merge-Sort.
How Does Swap Sort Work?
Here’s a step-by-step breakdown of how the swapsort algorithm works:
- Start at the beginning of the array: Begin by looking at the first pair of adjacent elements in the list.
- Compare the pair of elements: If the first element is larger than the second, swap them.
- Move one step forward: After swapping (or not, if they are already in the correct order), move to the next pair of elements.
- Repeat the process: Continue comparing adjacent pairs and swapping them if necessary, until you’ve processed the entire array.
- Repeat the pass: After a full pass through the list, repeat the process until no more swaps are needed, meaning the list is sorted.
Example
Consider an example list of numbers: [5, 2, 9, 1, 5, 6]
.
- First pass:
- Compare 5 and 2, swap them →
[2, 5, 9, 1, 5, 6]
- Compare 5 and 9, no swap →
[2, 5, 9, 1, 5, 6]
- Compare 9 and 1, swap them →
[2, 5, 1, 9, 5, 6]
- Compare 9 and 5, swap them →
[2, 5, 1, 5, 9, 6]
- Compare 9 and 6, swap them →
[2, 5, 1, 5, 6, 9]
- Compare 5 and 2, swap them →
- Second pass:
- Compare 2 and 5, no swap →
[2, 5, 1, 5, 6, 9]
- Compare 5 and 1, swap them →
[2, 1, 5, 5, 6, 9]
- Compare 5 and 5, no swap →
[2, 1, 5, 5, 6, 9]
- Compare 5 and 6, no swap →
[2, 1, 5, 5, 6, 9]
- Compare 6 and 9, no swap →
[2, 1, 5, 5, 6, 9]
- Compare 2 and 5, no swap →
After several passes, the array becomes fully sorted: [1, 2, 5, 5, 6, 9]
.
Time Complexity
The time complexity of swap sort is O(n²), where n is the number of elements in the list. This is because, in the worst case, each element is compared with every other element. For every pass, you make n-1 comparisons, and the process is repeated n times. Therefore, it is inefficient for large datasets.
Space Complexity
The space complexity of swap sort is O(1), meaning it requires only a constant amount of additional memory. Since it is an in-place sorting algorithm, it doesn’t need extra space for temporary arrays or additional data structures. It only requires a small amount of space for swapping elements.
Comparison
Case | Time Complexity | Space Complexity |
---|---|---|
Best Case | O(n²) | O(1) |
Average Case | O(n²) | O(1) |
Worst Case | O(n²) | O(1) |
Advantages
- Simple to implement: Swap sort is one of the easiest sorting algorithms to understand and implement, making it a good educational tool.
- In-place sorting: It doesn’t require extra space, which can be beneficial when memory usage is a concern.
Disadvantages
- Inefficient for large datasets: Due to its O(n²) time complexity, it becomes slow as the number of elements increases.
- Not stable: While not always the case, many implementations of this sort may not maintain the order of equal elements, which can be important for certain applications.
When Should You Use Swap Sort?
This sort is generally not the best choice for sorting large datasets, especially when faster algorithms like Quick Sort or Merge Sort are available. However, swap sort can be useful in cases where:
- The dataset is small.
- Memory usage is a critical factor, and you cannot afford to use extra memory.
- You need a very simple algorithm for educational purposes or basic applications.
Real-world applications of SwapSort
Here are the real-world applications
- Educational Tool: Used to teach basic sorting concepts and algorithm design due to its simplicity.
- Small Datasets: Suitable for sorting small datasets where performance is not a major concern.
- Embedded Systems: Can be used in systems with limited memory where efficiency is less critical.
- Low-Complexity Tasks: Useful in situations where quick implementation is needed for sorting small-scale data.
- Microcontroller-Based Applications: Ideal in environments where memory usage is minimal, and the dataset is small enough to make Swap Sort feasible.
Conclusion
Swap Sort is a basic sorting algorithm that works by repeatedly swapping adjacent elements until the entire array is sorted. While it is simple and easy to understand, its O(n²) time complexity makes it impractical for large datasets. If you’re dealing with small arrays or teaching sorting concepts, swap sort might be a good choice. However, for larger datasets, more efficient algorithms should be considered.
FAQs
Q1: What is the difference between Swap Sort and Bubble Sort?
Both Swap Sort and Bubble Sort work by repeatedly swapping adjacent elements that are out of order. However, Bubble Sort usually continues until the entire list is sorted, while SwapSort might be stopped early if no swaps are needed in a pass. Despite these differences, both algorithms have a time complexity of O(n²).
Q2: Why is Swap Sort inefficient for large datasets?
This Sort compares each element to every other element in the list, resulting in O(n²) time complexity. This makes it inefficient for large datasets, as the number of comparisons grows quickly as the size of the input increases.
Q3: Is Swap Sort stable?
No, Swap Sort is generally not considered stable. In its basic form, it may not maintain the relative order of equal elements, unlike algorithms like Merge Sort, which is stable.
Q4: Can Swap Sort be used in practical applications?
It is rarely used in practical applications due to its inefficiency. More advanced algorithms like Quick Sort, Merge Sort, or Heap Sort are preferred because of their better performance on larger datasets.
Q5: How do I implement Swap Sort in Python?
Here’s a simple implementation in Python:
def swap_sort(arr):
n = len(arr)
for i in range(n):
for j in range(n - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
# Example usage
arr = [5, 2, 9, 1, 5, 6]
print(swap_sort(arr))