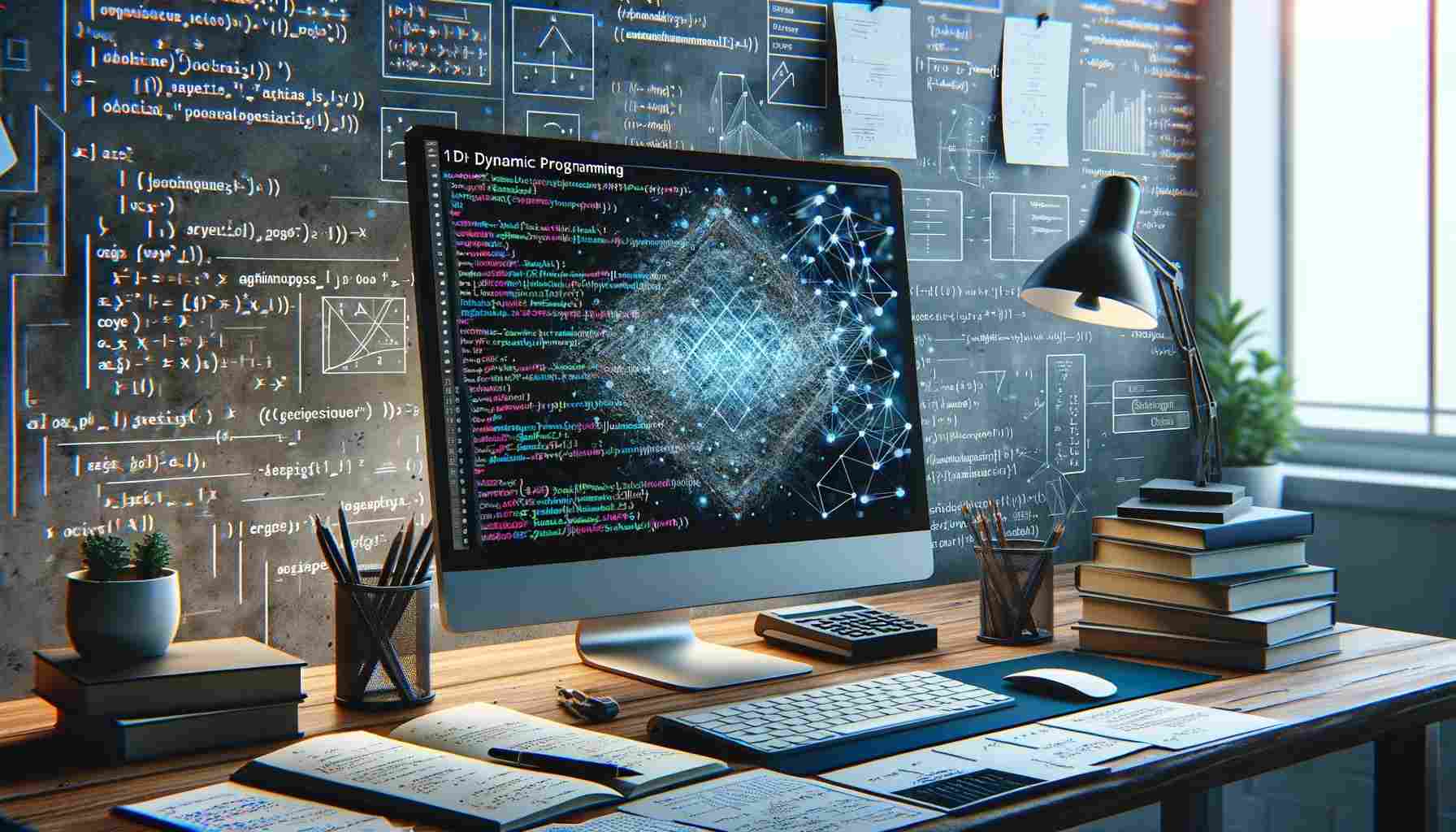
Dynamic programming is a problem-solving approach that has gained immense popularity in computer science due to its efficiency in solving complex problems. Among its various forms, 1D dynamic programming focuses on solving problems with a single dimension, such as those involving linear sequences or lists. This article provides essential tips and tricks for programmers seeking to master 1D dynamic programming, covering the fundamentals, common patterns, and best practices. Whether you’re preparing for coding interviews or working on algorithmic challenges, these insights will help you excel in using this powerful technique.
Understanding 1D Dynamic Programming
Dynamic programming involves breaking down complex problems into simpler subproblems and solving them optimally to build a solution to the original problem. In 1D dynamic programming, the problem structure is typically linear, with a single variable or index determining the state transitions. This linearity allows for a straightforward approach to solving the problem.
Key Concepts of 1D Dynamic Programming
- State Definition: The first step in 1D dynamic programming is defining the “state.” This involves identifying the parameter or index that determines the subproblem. The state often represents a position in a sequence or a specific decision point.
- Recursive Relation: The next step is to establish the recursive relation or “transition function.” This defines how the solution to a subproblem relates to other subproblems. In 1D dynamic programming, the recursive relation is typically linear, involving neighbouring indices or states.
- Base Cases: Identifying the base cases is crucial, as they serve as the starting point for building the solution. Base cases are usually straightforward and represent the simplest subproblems.
- Memoization: To avoid redundant calculations, memoization is used to store previously computed results. This is a key component of dynamic programming, allowing the algorithm to be more efficient.
Tips and Tricks for 1D Dynamic Programming
- Identify the Problem Structure: The first step in applying 1D dynamic programming is to understand the problem’s underlying structure. Look for patterns involving sequences, arrays, or linear relationships. Problems with a clear progression from one state to another are ideal candidates for this technique.
- Start with Small Examples: Before diving into complex problems, start with simpler examples to understand the recursive relation and state transitions. This helps build intuition and reveals the underlying patterns.
- Think Iteratively: While dynamic programming is often introduced with a recursive perspective, thinking iteratively can be more intuitive for 1D problems. Consider using loops to build the solution from the base cases to the desired outcome.
- Use a Table or Array: In 1D dynamic programming, an array or table is typically used to store the computed results. This structure allows for quick access to previously computed values and helps maintain the linearity of the solution.
- Optimize Space Complexity: Depending on the problem, you can optimize space complexity by reducing the size of the memoization structure. For example, if the recursive relation only depends on a limited number of previous states, you can use a sliding window or circular buffer to store results.
- Test with Edge Cases: When working with 1D dynamic programming, it’s crucial to test the solution with edge cases. This helps ensure that the base cases are correctly defined and that the recursive relation handles all possible transitions.
- Understand the Time Complexity: Dynamic programming is known for its efficiency, but it’s essential to understand the time complexity of the solution. Analyze the number of iterations and recursive calls to ensure the solution is optimal.
Common Patterns in 1D Dynamic Programming
- Fibonacci Sequence: A classic example of 1D dynamic programming is the Fibonacci sequence, where each term is the sum of the previous two terms. This pattern demonstrates the linearity and recursive relation typical of 1D problems.
- Longest Increasing Subsequence: This pattern involves finding the longest subsequence in an array where elements are in increasing order. It showcases the iterative approach and memoization used in 1D dynamic programming.
- Knapsack Problem (1D Variant): While the knapsack problem is often associated with 2D dynamic programming, a 1D variant involves optimizing a sequence of items to maximize value without exceeding a weight limit. This pattern illustrates the importance of state definition and recursive relation.
Conclusion
1D dynamic programming is a versatile and powerful technique for solving a wide range of problems involving linear sequences and states. By understanding the key concepts and applying these tips and tricks, programmers can master this approach and solve complex algorithmic challenges with ease. Whether you’re preparing for coding interviews or working on real-world projects, mastering 1D dynamic programming is a valuable skill that can significantly enhance your problem-solving capabilities.